Embed API
Embed API
The system allows users to directly write JavaScript code in the editor, which serves as a built-in interface to provide personalized functionality for the questionnaire. The built-in interface requires a name to be assigned and a piece of JavaScript code to be written.
Generally, when the amount of code is small, the EMBED method can be used to achieve the same effect as the FAKE method, and it is simpler. When the amount of code is large, the FAKE method is more convenient for maintenance. After the questionnaire goes live, the EMBED method requires the questionnaire to be stopped before modifications can be made, whereas the FAKE method does not have this restriction.
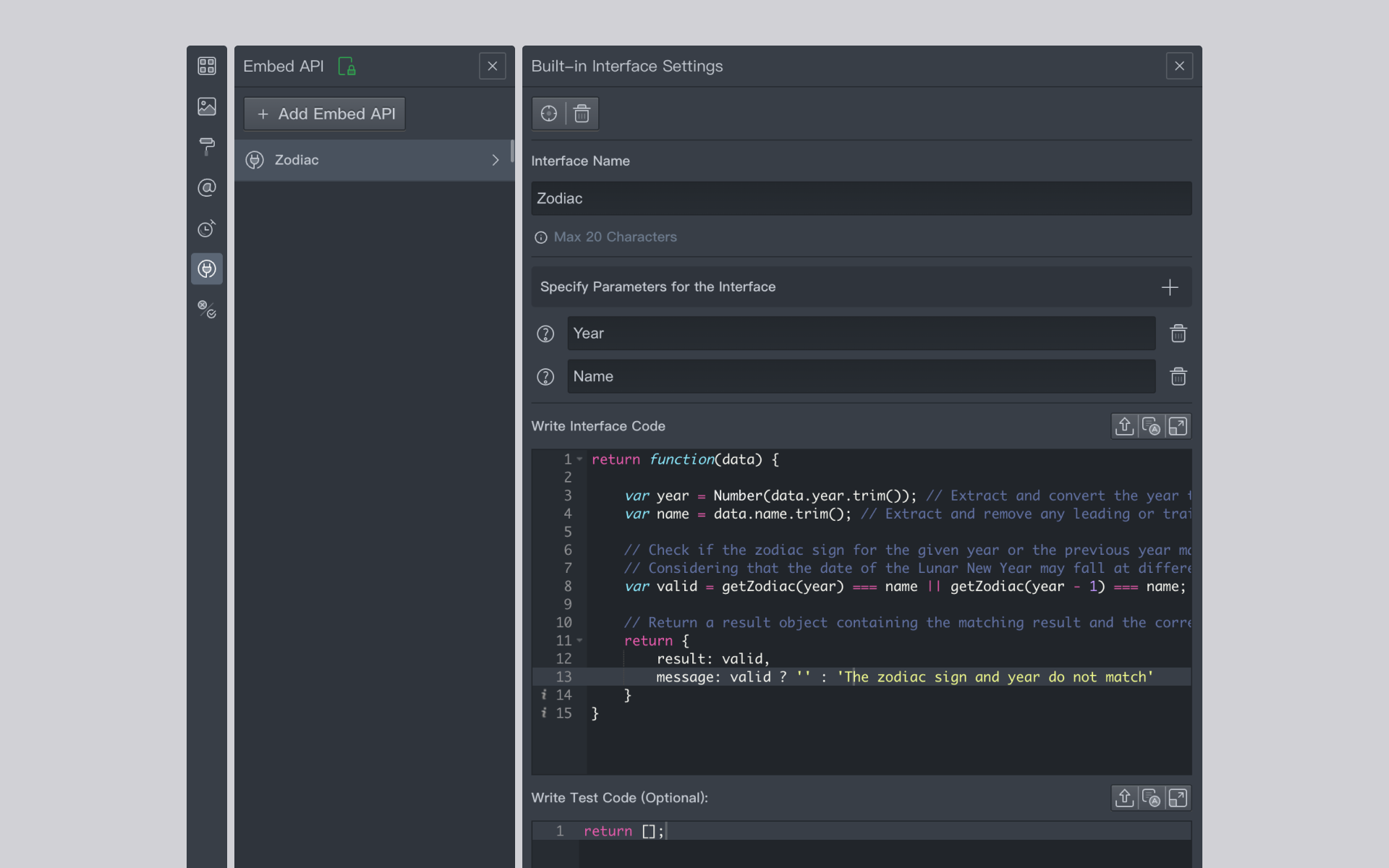
The embed API panel displays all the embed APIs for the questionnaire. If there is a yellow small triangle icon in the top right corner of a embed API's name, it indicates that the API has been used by a question node. If the small triangle icon is semi-transparent, it means that although the API is used by a question node, that node is not connected in the questionnaire.
If it is detected that the questionnaire uses unsafe scripts in the EMBED API method, or if the questionnaire receives complaints from respondents, customer service may remove it from the whitelist at any time without prior notice, and the account may be disabled.
Interface Name
The name of the embed API.
Parameters
Specify the parameters for the API. After specifying the parameter names, when using this embed API in the API request module, values must be passed for all parameters.
Interface Code
The javascript code written according to requirements.
The API code must ultimately return a function as the API processing function, and the return value of this function must be an object containing the properties result
and message
.
Case Study
When given a specific year and a corresponding zodiac sign, we wish to determine whether the two match correctly.
Therefore, this API will require two parameters:

year
parameter: Represents the year.name
parameter: Represents the name of the Chinese zodiac.
After determining the parameters, write the following code to make the determination.
var zodiacs = ['Mouse', 'Ox', 'Tiger', 'Rabbit', 'Dragon', 'Snake', 'Horse', 'Goat', 'Monkey', 'Chicken', 'Dog', 'Pig'];
// Define a function to get the zodiac sign corresponding to a given year
var getZodiac = function (year) {
// The Chinese lunar zodiac signs repeat every 12 years, and the corresponding zodiac sign is determined by taking the remainder of the year.
// However, due to the fact that the starting year of the zodiac signs (such as the Jiazi year corresponding to the Year of the Mouse) does not fully correspond to the Gregorian calendar year,
// a simple adjustment method is adopted here: process the remainder to match the actual zodiac sign.
// Note: The processing method here is a simplified example and does not accurately reflect the conversion between the lunar and Gregorian calendars.
var extra = year % 12;
extra = extra < 4 ? 12 - 4 + extra : extra - 4;
return zodiacs[extra];
};
// Return a function that takes a data object as a parameter and checks if the year matches the zodiac sign.
return function(data) {
var year = Number(data.year.trim()); // Extract and convert the year to a number.
var name = data.name.trim(); // Extract and remove any leading or trailing whitespace from the zodiac sign name.
// Check if the zodiac sign for the given year or the previous year matches the provided zodiac sign name.
// Considering that the date of the Lunar New Year may fall at different times within the Gregorian calendar year, two years are checked here.
var valid = getZodiac(year) === name || getZodiac(year - 1) === name;
// Return a result object containing the matching result and the corresponding message.
return {
result: valid,
message: valid ? '' : 'The zodiac sign and year do not match'
}
}
After providing the test code, the system will automatically run the tests. If the result obtained by calling the API code with the parameters specified in the test code does not match the expected result, an error will be reported.
Using embed APIs
When using it, select a question node, then open the settings panel and set the custom validation method to EMBED. Configure the parameters and input option variables as required. When a respondent reaches this question, the embed API will be requested.
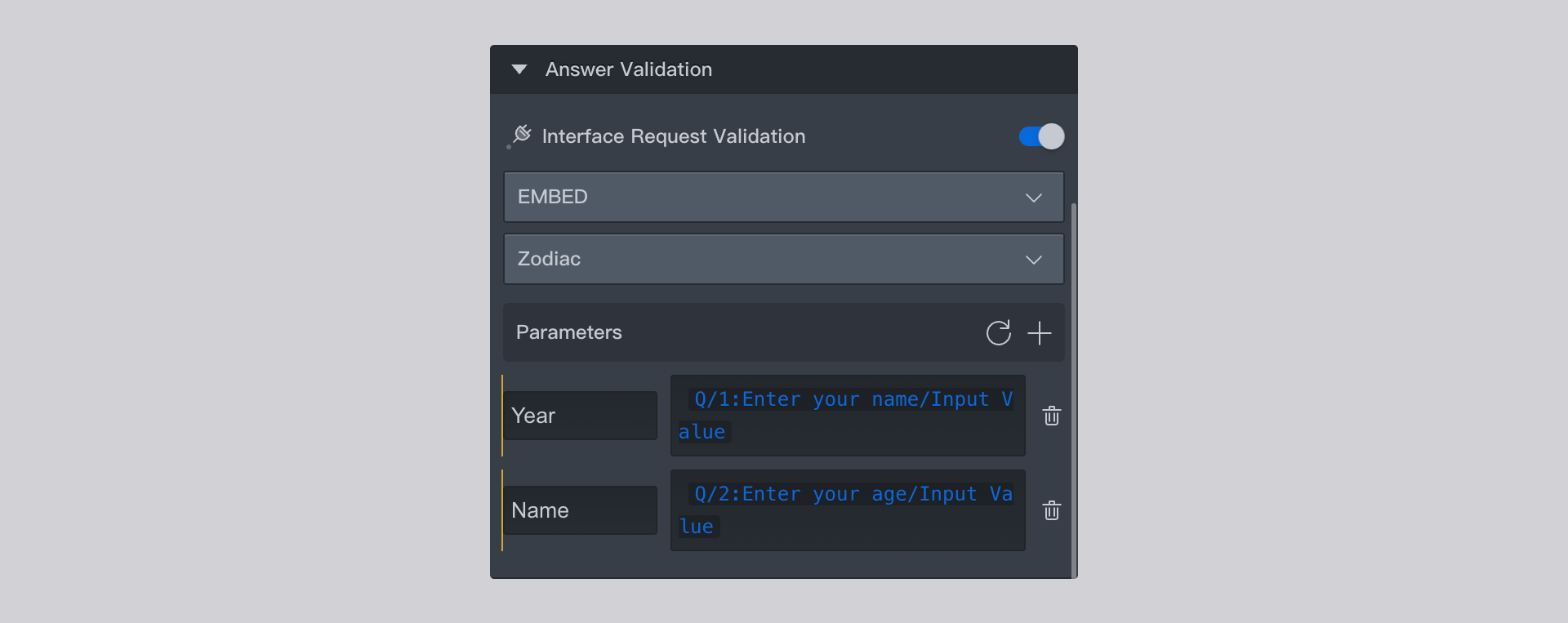