Global General Settings - Settings
Global General Settings - Settings
Settings
Survey External Actions
Trigger external actions outside the questionnaire, such as sending emails, sending SMS messages, and so on. Each question supports up to 10 external file actions.
Crearte New Action
Trigger Scenarios
- On Forward Entry: Triggers when entering the current question from the previous one.
- On Forward Leave: Triggers when moving from the current question to the next one.
- On Backward Entry: Triggers when returning to the current question from a previous one.
- On Backward Leave: Triggers when moving back from the current question to a previous one.
Type of Action
- Send Email: Sends an email to a specified email address. Requires setting the recipient's email address, email subject, and the email body text.
- Send SMS: Sends an SMS message to a specified phone number. Sending SMS requires inputting the specified SMS service API call interface and accessing the required parameters according to the API specifications.
- Invoke API: Calls a specified API, supporting four different methods: GET, POST, FAKE, and EMBED. By default, it sends via HTTPS.
- Condition: Sets logical judgment conditions. After setting logical judgment conditions, the specified action will only be triggered when the conditions are met upon encountering the trigger scenario.
Answer Validation
During the process of respondents filling out a questionnaire, it is often necessary to conduct multi-angle validation of the answers to ensure the quality of their responses. This includes checking for inconsistencies in answers, verifying whether responses match relevant records in the client's system, and so on. When logical judgment and validation of questionnaire answers are enabled, respondents will not be allowed to continue if issues in their answer logic are detected that prevent them from passing validation. This prevents the acceptance of invalid answers. If validation fails, the returned content can include an explanation of the reason for failure, which respondents can see in real-time during the answering process. The advantage of interface logic validation is its powerful functionality.
Interface Request Validation
Interface request validation is typically used for personalized validation and supports four different methods: GET, POST, FAKE, and EMBED. By default, requests are sent via HTTPS to an external validation interface with parameters, and the validation pass or fail is determined by examining the interface's response. If validation fails, the response can include an explanation of the reason for failure, which respondents can see in real-time during the answering process. The development of interface request validation interfaces requires basic programming skills, and its advantage is its powerful functionality.
Interface Request Methods
Interface request methods include: GET, POST, FAKE, and EMBED.
- GET: Requests a remote interface using HTTP GET, with parameters appended to the URL as a queryString.
- POST: Requests a remote interface using HTTP POST, with parameters placed in the body.
- FAKE: A custom request method.
- EMBED: A custom request method.
GET
Set the GET request method and fill in the request address. Then, configure and add the parameter names and values for the request.
During the answering process, whenever the questionnaire encounters a point where an interface request is required, it will send a request to the specified address with the parameters, expecting the interface to return data in the following format:
{
result: {string|number|boolean} // The calculation result. For verification interfaces, returning false indicates failure, while returning true indicates success. For non-verification interfaces, it returns a numerical value or string as the calculation result.
message?: string // For verification interfaces, if the result property is false (indicating verification failure), a verification failure message should be carried through the message property.
}
The message property of the returned result can also include formats with special meanings, and different formats will have different effects on the answer page:
- URLs starting with "https://": Upon receiving the returned result, the user will be redirected to this address, leaving the questionnaire.
- Messages starting with "vital:": Upon receiving the returned result, a message will pop up and terminate the answering process.
- Other messages: The message will be displayed in a sliding warning popup window.
POST
The setup is the same as for GET, with the only difference being the request method.
FAKE
A FAKE request invokes a pre-deployed JavaScript script on the server. This JavaScript script needs to be written according to specified specifications.
Below is an example illustrating how to achieve the following requirement through a request:
- Use the FAKE method to call the interface at
https://xxx.test.com/plugin/sum_gt0
, which requires two parameters,number1
andnumber2
. The purpose of this interface is to determine whether the sum of the two numbers is greater than 0. - Additionally, call the interface at
https://xxx.test.com/plugin/gt
, which also requires two parameters,number1
andnumber2
. The purpose of this interface is to determine whethernumber1
is greater thannumber2
.
- First, write a script according to the following specifications:
(function () {
// The first three lines are written this way, and this is a fixed rule.
var plugin = window.CFPlugin = window.CFPlugin || {};
var server = plugin.fakedServers = plugin.fakedServers || {};
var space = plugin.currentSpace; // This variable represents the current namespace or space.
/**
* Add a method to `server` according to the following rule. This method will be responsible for handling requests to the `sum_gt0` interface under this namespace.
* In other words, requests made to `https://xxx.test.com/plugin/sum_gt0` will actually invoke this method, which will receive and process the request.
*/
server[space + '/sum_gt0'] = function (data) {
// This uses the two properties `number1` and `number2` in `data` as parameters.
// The return format follows the specified rules above.
return {
result: Number(data.number1) + Number(data.number2) > 0, // Note: Corrected the addition to use Number() for both parameters.
message: 'message', // This is a placeholder message.
};
};
// Annotate the parameter specifications for this method in this way.
// However, there's a mistake here because the annotation should be for the correct method name.
// Corrected line should be:
server[space + '/sum_gt0'].params = ['number1', 'number2']; // Corrected the method name and added the correct parameters.
/**
* Add a method to `server` according to the following rule. This method will be responsible for handling requests to the `gt` interface under this namespace.
* In other words, requests made to `https://xxx.test.com/plugin/gt` will actually invoke this method, which will receive and process the request.
*/
server[space + '/gt'] = function (data) {
// This uses the two properties `number1` and `number2` in `data` as parameters.
// The return format follows the specified rules above.
return {
result: Number(data.number1) > Number(data.number2), // Note: Corrected the comparison to use Number() for both parameters.
message: 'message', // This is a placeholder message.
};
};
// Annotate the parameter specifications for this method in this way.
// Corrected line should be associated with the correct method name:
server[space + '/gt'].params = ['number1', 'number2']; // Added the correct parameters for the 'gt' method.
// Additional explanatory notes:
// Therefore, requests to `https://xxx.test.com/plugin/test_2` will be handled by the following method.
server[space + '/test_2'] = function (data) {
// Input code here.
// Specific rules are written inside this method.
// Placeholder return statement:
return {...}; // Replace `{...}` with the actual return object.
};
// If you want to fake the `https://example.cf.io/fake/test` interface,
// then you need to deploy a `fake.js` script to `https://example.cf.io` and write it according to the above format, with a 'test' method included.
})();
-
Deploy the script onto the server and ensure that it can be accessed via the URL
https://xxx.test.com/plugin.js
. -
Set the request method to FAKE, fill in the address as
https://xxx.test.com/plugin/sum_gt0
, and add two parameters,number1
andnumber2
, each with a specified value. For another interface, fill in the address ashttps://xxx.test.com/plugin/gt
, and specify the parameters following the same rules. The address is essentially the location of the script with the.js
suffix removed, followed by the corresponding method name. -
Since the script will be deployed on the user's own server, it will not be on the system's trusted whitelist by default. In this case, you can contact our customer service, who will add the script's location to the system whitelist. The system will refuse to load scripts from addresses not on the whitelist. If unsafe code is detected in the script or if complaints are received from survey respondents, customer service may remove it from the whitelist at any time without prior notice. The account may be disabled as a result.
-
If the above steps are completed correctly, when the survey reaches the point of using this request, it will execute the specified method in the script and obtain the final calculation result.
Generally speaking, the FAKE interface is recommended for very complex calculations or personalized scenarios with low security requirements. For scenarios with higher security requirements, it is recommended to use GET or POST interfaces.
EMBED
Also known as the built-in interface, it is used for writing and managing built-in interface code, allowing direct javascript code writing in the editor to achieve the same effect as the FAKE method, but in a simpler way. When selecting the EMBED method, a dropdown list will be displayed, where you can choose previously added built-in interfaces. Generally, the EMBED method is used when the amount of code is small. When the code volume is large, the FAKE method is more convenient for maintenance. After the survey is launched, modifications using the EMBED method require stopping the survey first, while the FAKE method does not have this restriction.
If it is detected that an insecure script is used in the EMBED method of a survey, or if complaints are received from survey respondents, customer service may remove it from the whitelist at any time without prior notice. The account may be disabled.
To add a built-in interface, open the built-in interface panel and click the "Add" button.
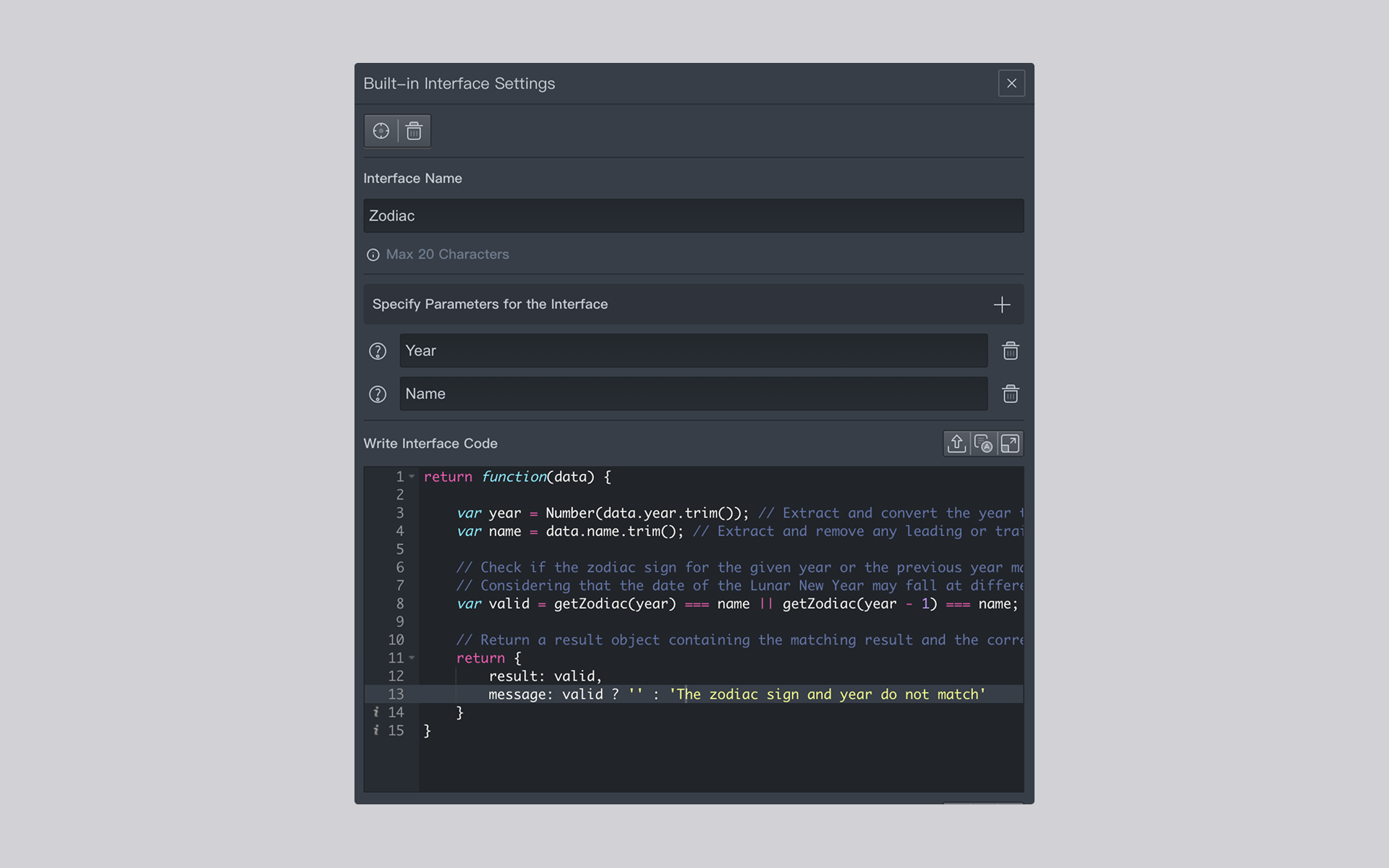
A built-in interface requires specifying a name and a piece of javascript code, with the option to add parameters and test sample code. The built-in interfaces of the survey will be displayed in the built-in interface list. If a yellow small triangle icon appears in the top right corner of a built-in interface name, it indicates that the interface has been used by a question node; if the small triangle icon is semi-transparent, it means that although the interface is used by a question node, that node is not connected to the survey.
Interface Name
The name of the interface, which can be selected by name in the interface request module to use the built-in interface.
Parameters
Specify the parameters of the interface. After specifying the parameter names, when using this built-in interface in the interface request module, values must be passed for all parameters. The interface code can obtain the actual parameters from the first parameter of the interface processing function by accessing its properties.
Interface Code
Write javascript code according to business requirements. The interface code must return a function as the interface processing function, and the return value of this function must be an object containing result
and message
properties.
After understanding the coding rules, let's look at a practical example: Given a specific year and a Chinese zodiac name, we want to determine if they match correctly. Therefore, this interface will require two parameters:

year
parameter: Represents the year.name
parameter: Represents the name of the Chinese zodiac.
After determining the parameters, write the following code to make the determination.
var zodiacs = ['Mouse', 'Ox', 'Tiger', 'Rabbit', 'Dragon', 'Snake', 'Horse', 'Goat', 'Monkey', 'Chicken', 'Dog', 'Pig'];
// Define a function to get the zodiac sign corresponding to a given year
var getZodiac = function (year) {
// The Chinese lunar zodiac signs repeat every 12 years, and the corresponding zodiac sign is determined by taking the remainder of the year.
// However, due to the fact that the starting year of the zodiac signs (such as the Jiazi year corresponding to the Year of the Mouse) does not fully correspond to the Gregorian calendar year,
// a simple adjustment method is adopted here: process the remainder to match the actual zodiac sign.
// Note: The processing method here is a simplified example and does not accurately reflect the conversion between the lunar and Gregorian calendars.
var extra = year % 12;
extra = extra < 4 ? 12 - 4 + extra : extra - 4;
return zodiacs[extra];
};
// Return a function that takes a data object as a parameter and checks if the year matches the zodiac sign.
return function(data) {
var year = Number(data.year.trim()); // Extract and convert the year to a number.
var name = data.name.trim(); // Extract and remove any leading or trailing whitespace from the zodiac sign name.
// Check if the zodiac sign for the given year or the previous year matches the provided zodiac sign name.
// Considering that the date of the Lunar New Year may fall at different times within the Gregorian calendar year, two years are checked here.
var valid = getZodiac(year) === name || getZodiac(year - 1) === name;
// Return a result object containing the matching result and the corresponding message.
return {
result: valid,
message: valid ? '' : 'The zodiac sign and year do not match'
}
}
Test Code
To ensure that the written code is free of errors or will not be inadvertently modified incorrectly in subsequent changes, several test case codes can be added. The test case codes need to return an array, with each item in the array representing a test case. If you do not wish to provide test codes, you must use the default generated test codes; the test codes cannot be empty.
Below, we will add test codes to the above example of zodiac sign validation, and provide explanations in the code comments.
javascript
return [
{
params: {
year: '1989',
name: 'snake'
},
result: true // 1989 is the Year of the Snake, so the result should be true.
},
{
params: {
year: '1989',
name: 'Mouse'
},
result: false // 1989 is not the Year of the Rat, so the result should be false.
},
{
params: {
year: '1991',
name: 'Goat'
},
result: true // 1991 is the Year of the Goat (or Sheep, depending on the cultural context), so the result should be true.
}
];
If test codes are provided, the system will automatically run the tests. An error will be reported if the result obtained by calling the interface code with the specified parameters in the test codes does not match the expected result.
Using the EMBED Interface
When using it, select a question node, then open the settings panel and set the custom validation method to EMBED. Configure the required parameters and input option variables as instructed. When a respondent encounters this question, it will trigger a request to the embedded interface.
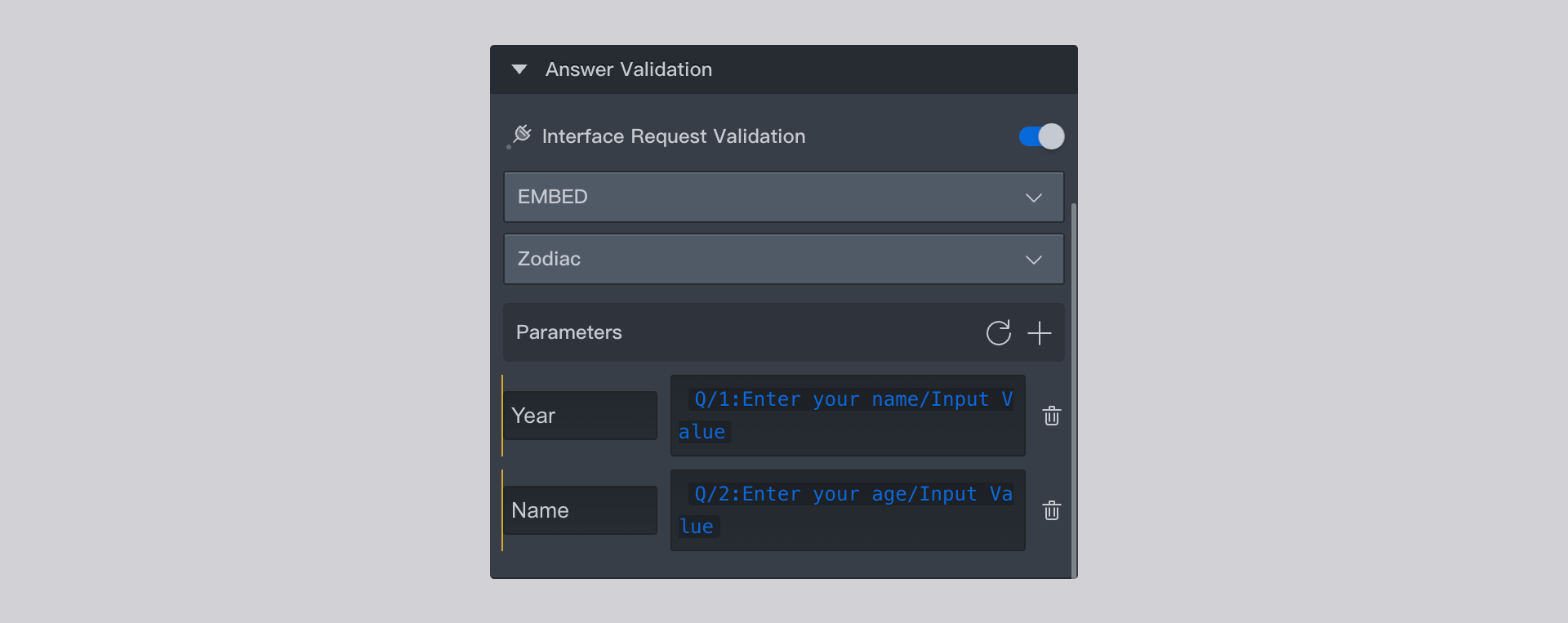
Built-in Logic Validation
Although interface request validation is powerful, it requires developing an external interface. Enabling built-in logic validation allows you to use the system's logical operation tools to achieve the function of interface request validation without needing to develop an external interface. The advantage is that it is simpler to implement.
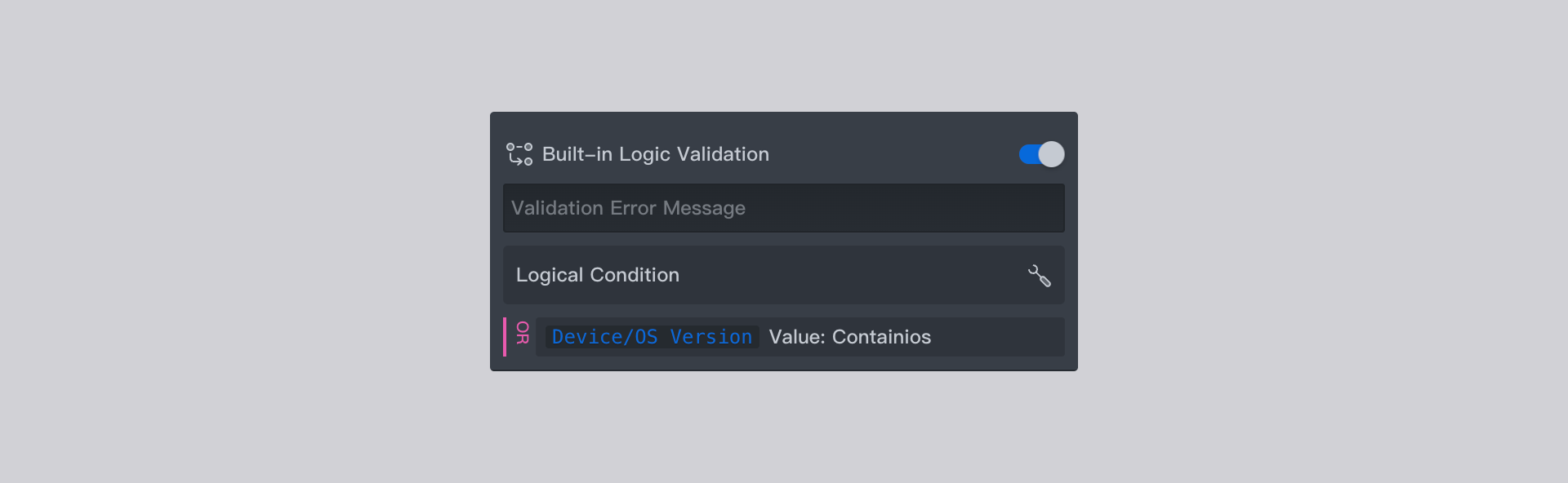
Validation Settings
Allow Continuing on Validation Failure
By default, respondents are not allowed to continue answering questions if validation fails. When this option is enabled, respondents are allowed to continue answering questions even if validation fails.
Question Backtracking Settings
When a validation issue is detected during the respondent's questionnaire completion, they can be forced to return to a specified question and answer correctly. The usage method is similar to the validation settings feature.
API Request Judgment
An external custom interface is used to verify and judge whether the respondent has answered correctly.
Built-in Logic Judgment
Internal logic settings are used to verify and judge whether the respondent has answered correctly.
When both interface request judgment and built-in logic judgment are set, the system will first perform interface request judgment and then built-in logic judgment.
- When the interface returns a rollback permission, it will determine whether to rollback based on the result of the built-in logic judgment.
- When the interface returns a no-rollback permission, regardless of the result of the built-in logic judgment, a rollback cannot be performed.
Return to Question When Above Conditions Are Met
Specify a question in the dropdown list. When the rollback trigger conditions are met, the questionnaire will jump to this specified question.
Advanced Settings
Hidden Question
When a question is set as a hidden question, it can be used like a normal question, but it is invisible to respondents. It is often used for initial data cleaning and data labeling through automatic selection and filling during questionnaire responses. Hidden questions can also be referenced by subsequent questionnaire questions and can participate in variable calculations.
Show When Only One Reference Option Exists
Sometimes a questionnaire question may have only one option. For example, if a respondent selects an option on one question, the options for another subsequent question may come from the respondent's previous selection. In this case, the subsequent question will have only one option. At this point, you can decide whether to present this question based on your needs.
- No: Do not show it to the respondent and automatically select the option.
- Yes: Still show it to the respondent.
- When Conditions Are Met: Show it to the respondent only when the logical condition settings are met.